Improve Entity Framework Performance with NGen
In another post, I introduced very quickly about NGen, which serves to pre-compile an application's assemblies, doing the work of JIT (Just in Time Compiler) in advance.
Just to remind you a little, the figure below shows the process of running an application in .NET:
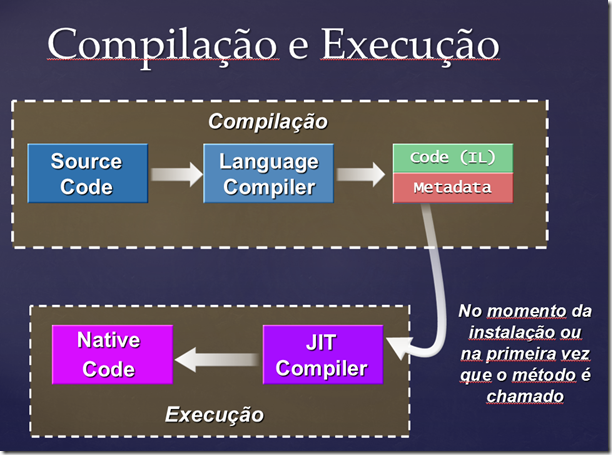
In a natural process of development in .NET, what happens is basically what is in the figure above, you write your code, compile it, and then execute it. But in execution, JIT comes into play, which takes the generated code (Assembly) and translates it into native code, or machine language, which will then be executed by the processor.
During the installation of the .NET Framework on a computer, this process is performed for all .NET components. The .NET core is automatically precompiled on your computer, but all the code you produce does not go through this process.
As I mentioned in the previous post, since version 6, Entity Framework works separately from .NET, through EntityFramework.dll
, which is usually downloaded by NuGet inside Visual Studio. This process simply downloads the DLL from the NuGet repository and copies it to a folder in your project. That is great and works great!
Many people complain on forums and blogs about the initial load time of the Entity Framework, when the first command executed, whatever it may be, takes a while, but all subsequent instructions are very quick. Of course, the design of the model and the command can influence this time, but one thing that affects a lot is the lack of precompilation.
Let's see a straightforward example. I will create a Console project in C # and do reverse engineering using the Entity Framework Power Tools, which I have already explained in a previous post. So to accompany me, create a Console type project in Visual Studio, as shown in the figure below:
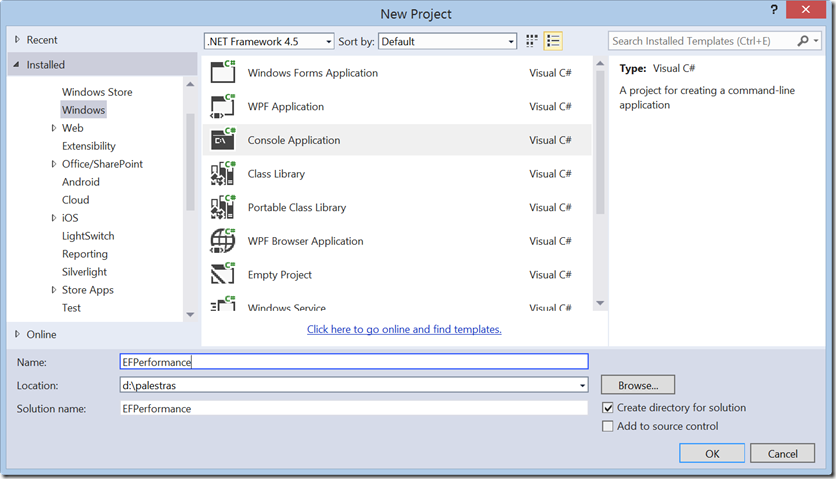
Now let's reverse engineer the Northwind
database, but you can use any other database you have.
PS: Don't forget to install EntityFramework using NuGet: Install-Package EntityFramework
.
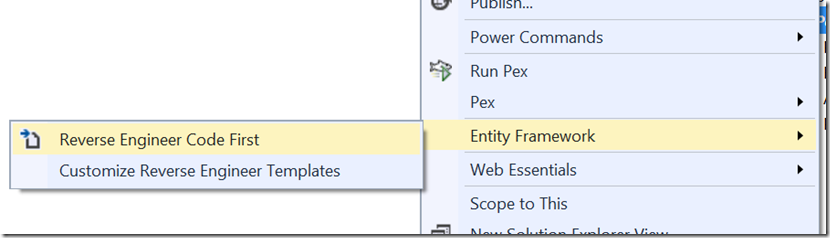
Now I will choose the database and generate the classes.
To show the difference in performance, I will create a simple query in EF, and to mark the time, we will use the class StopWatch (). See below:
static void Main(string[] args) { Stopwatch clock = new Stopwatch(); clock.Start(); var db = new NorthwindContext(); var datas = db.Customers; foreach(var c in datas) { Console.WriteLine(c.CompanyName); } clock.Stop(); Console.WriteLine("\n\nQuery Time: {0} segs.\n\n", clock.Elapsed.TotalSeconds); }
See the result:
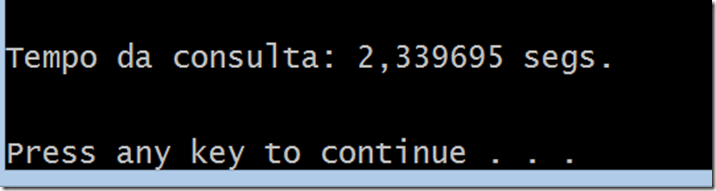
Now we are going to use NGen in this project and retake the test. To do this, open a Visual Studio command prompt (as an administrator) and run the command below:
ngen Install EFPerformance.exe
You see something like this:
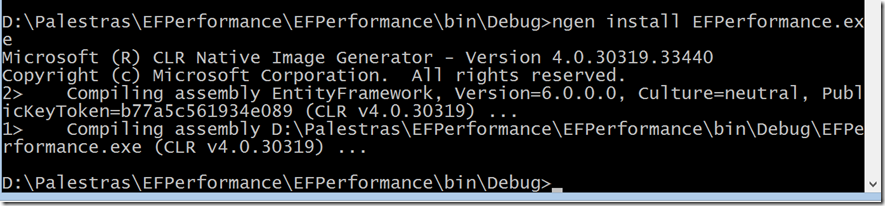
Notice that in addition to our project (EFPerformance.exe
) the EntityFramework dll was also compiled.
Now let's run the program again, straight from the command line and look at the speed difference:
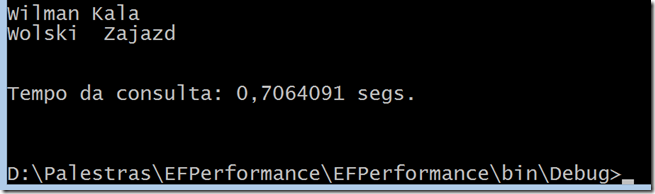
Realize that it got extremely faster !!!
Now, if you have a web application, you can improve performance using the command of aspnet_compiler. Still, it does not generate native images like NGen, despite increasing performance by precompiling some project files (.aspx
, .ascx
, app_code
, etc.). If you prefer, the Visual Studio IDE has this option when publishing the web application:
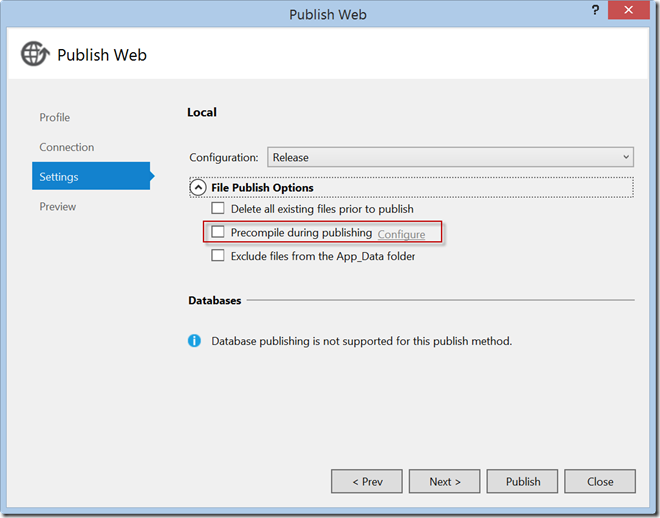
I hope you liked the tips!