Reverse Engineering Tables and Views with EF Code First
Some time ago, I made a post talking about how to take advantage of your existing database with EntityFramework Power Tools, but the Power Tools model does not allow you to choose the tables or views you want to read, and sometimes this is very useful.
With the release of Visual Studio 2013 Update 2, and also EntityFramework 6.1, a new option was added in the data sources. It now allows making reverse engineering
with Code First to choose which tables and views will be read, similar to what was already possible with the EntityFramework Designer.
To demonstrate this, let's create a new Console project in Visual Studio 2013:
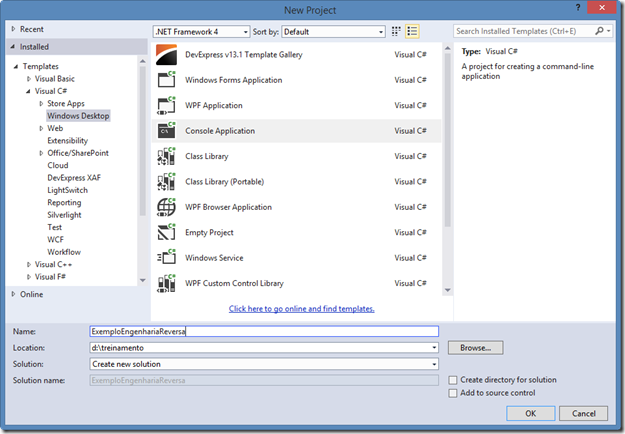
Now we are going to do the reverse engineering, but using the new feature of Visual Studio 2013. To do this, in your project, right-click on the solution and then go to Add -> New Item:
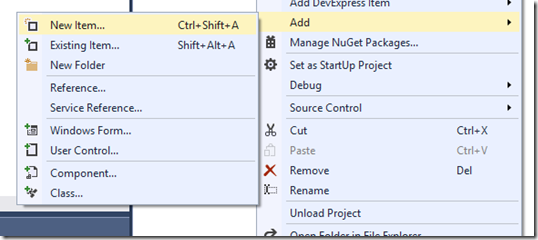
Now let's add an ADO.NET Entity Data Model:
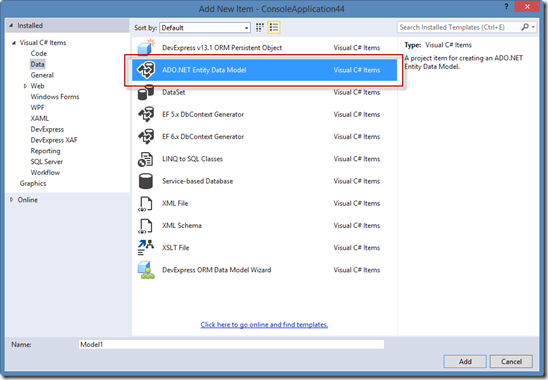
And notice that there is now a new option called "Code First from database" and with it, we will read an existing database and create all the code for EF Code First.
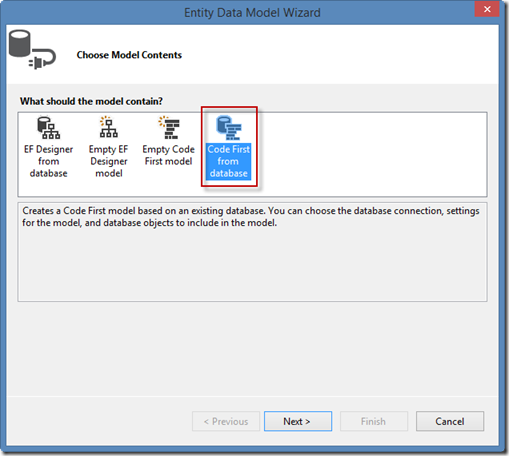
We will then choose this option and place the connection to our existing database: (in my example I am using the Microsoft database called Northwind
)
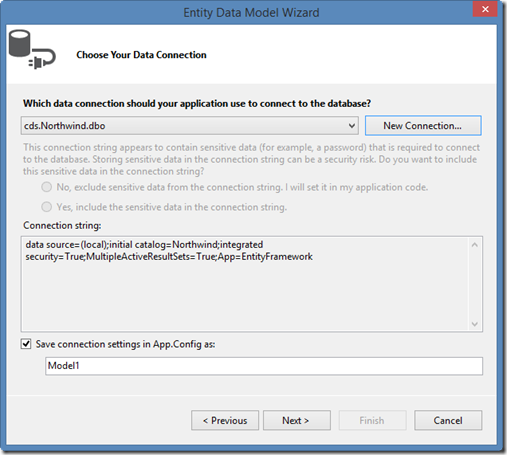
Now you can choose the tables and views you want for your Code First project:
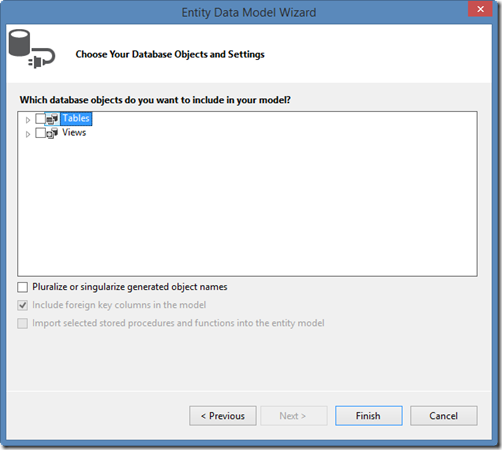
All classes will be generated in Code First, but with a big difference with Power Tools: the mapping information is generated with Data Annotations instead of Fluent API. See the example of the Product
class:
public partial class Product { [Key] public int ProductID { get; set; } [Required] [StringLength(40)] public string ProductName { get; set; } public int? SupplierID { get; set; } public int? CategoryID { get; set; } [StringLength(20)] public string QuantityPerUnit { get; set; } [Column(TypeName = "money")] public decimal? UnitPrice { get; set; } public short? UnitsInStock { get; set; } public short? UnitsOnOrder { get; set; } public short? ReorderLevel { get; set; } public bool Discontinued { get; set; } public virtual Categories Categories { get; set; } }
See that the class was generated, and Data Annotations was created.
Now just use EntityFramework Code First!