Using Entity Framework Power Tools for Code First Development
When a company decides to adopt an ORM tool (Relational Object Mapping - in our case Entity Framework), a big paradigm break begins, as you will no longer access the database directly (Select
, Insert
, Update
, Delete
, etc.), and will start to work only with classes and objects. That makes development much easier and more productive since you don't have to worry about the commands that go to the database, as this is the ORM's job.
But what about when you already have a database ready? That always generates another discussion. After all, you can build a new database, take advantage of the process and improve everything, or reuse the existing database structure and include only the ORM? You want to hear something very common: you are creating a new functionality or a new project, and you decide to use Entity Framework, but you need to read and write data from the current database. How to make it? Write the POCO classes by hand? Obviously not, because for this, several tools can do this for you, and one that we are going to present today is Entity Framework Power Tools.
To get started you need to download Power Tools using Visual Studio extensions management (Tools / Extensions and Updates), and then search for the Entity Framework Power Tools, as shown in the figure below:
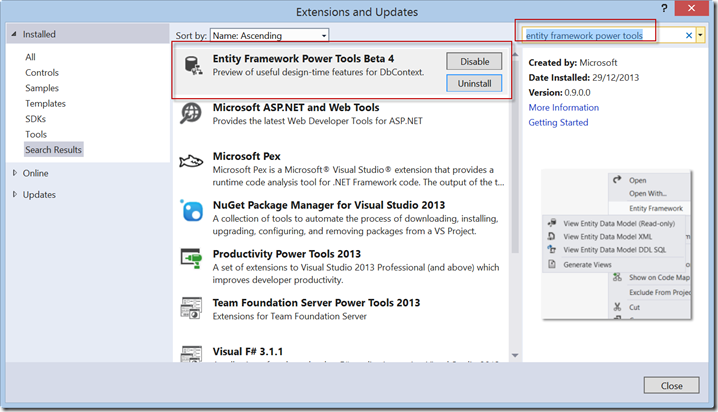
In my case, it is already installed. But, if yours is not already, click the "Install" button and you're done! Now let's create a project to explore the features.
Let's start by creating a Console project and adding Entity Framework through NuGet:
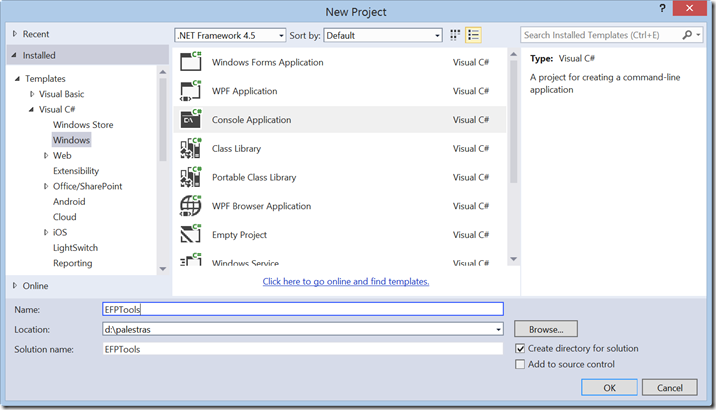
Now let's add EF:
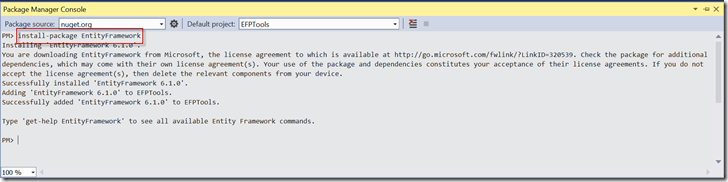
Now that we have Entity Framework, let's check in the menu of our solution a new item called Entity Framework (to see this menu, right-click on the name of your project):

See that we have two options:
Reverse Engineer Code First: allows you to read an existing database and generate the classes and the context;
Customize Reverse Engineer Templates: allows changing the generation format of the classes through a T4 file.
If you choose to customize the templates, you will have the following files added to the project, which can be modified according to your needs:
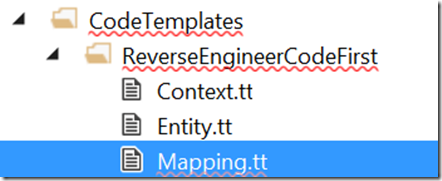
Choosing to generate the classes with the option "Reverse Engineer Code First" you will have a screen to put the access data to your database, do this and then click OK to start the generation of the classes:
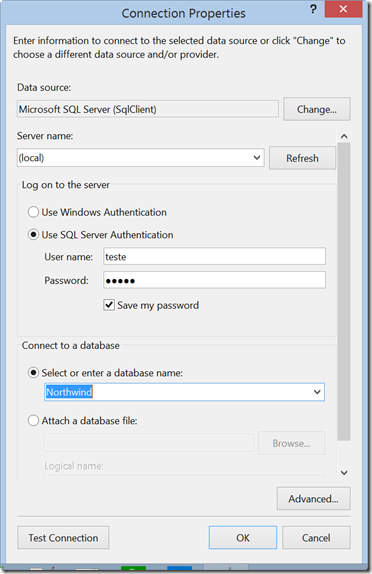
Now we have the entire database mapped, and in the current version of the Entity Framework, this includes tables and views. See a part of these classes in our solution:
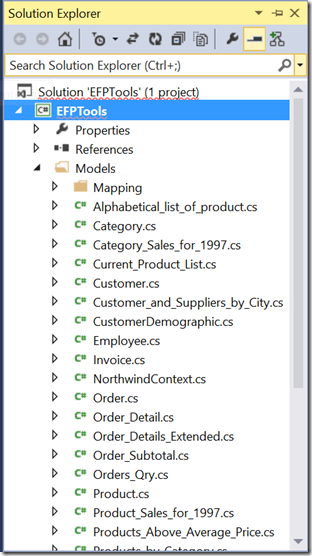
Now let's right-click on our context: NorthwindContext.cs
:

See that we now have other options, which are related to the database that was mapped. As we are working with Code First, we do not have a visual diagram of our database. But with the help of Power Tools, by clicking on the first option: View Entity Data Model (Read-only), you can graphically visualize how your database is doing. See below:
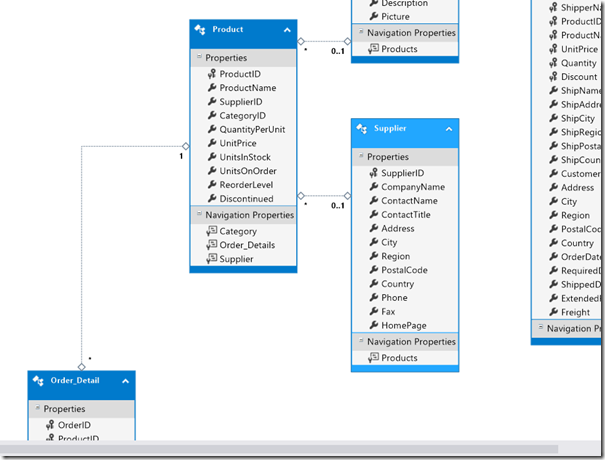
See that all relationships have been created and are generated through Fluent API in the mapping file. Note that the menu reads "Read-only" and this means that this diagram is for reading only, so there is no point in writing anything in it that will not be reflected in the entities.
It is also possible to generate an XML file, which is similar to the EDMX file (Model First and Database First). There is also an option to generate a DDL script, which can be executed on the database server to create it. In the case of Entity Framework Code First, there is a migration feature that eliminates this work of creating and updating the database. See this post here.
Finally, we have a very interesting option: "Generate Views" that generates queries optimized for the database and helps to improve the performance of our application. Remembering that if you choose to use Views, you should generate them whenever you modify your classes.
I hope I have clarified the use of the Entity Framework Power Tools and that this article will be very useful for you!